DOM 문서 객체 모델
- DOM(Document Object Model) 의 정의
- 넓은 의미 : 웹 브라우저가 HTML 페이지를 인식하는 방식
- 좁은 의미 : Document 객체와 관련된 객체의 집합
- HTML 태그를 추가, 수정, 삭제 할 수 있다.
- DOM의 계층 구조
- Element 객체 : 태그
- Text 객체 : 태그 안의 내용
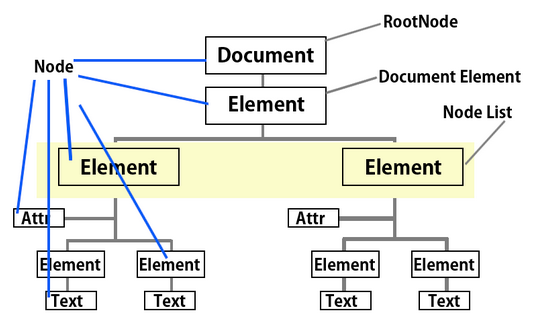
DOM 메소드
- getElementById(id) : ID를 탐색하여 하나의 문서 객체 반환
⭐ ID는 유일한 값을 가지므로 하나의 객체만 반환됨
window.onload = function() {
var header1 = document.getElementById('header_1');
var header2 = document.getElementById('header_2');
// 태그 안에 삽입
header1.innerHTML='별';
header2.innerHTML='달';
};
<body>
<h1 id="header_1"></h1>
<h1 id="header_2"></h1>
</body>
- getElementsByTagName(tag) : 태그명을 탐색하여 문서 객체의 배열 반환
// body태그를 먼저 읽기위해 자바스크립트를 지연시킴
window.onload = function() {
var spans = document.getElementsByTagName('span');
// 태그 안에 삽입
spans[0].innerHTML = '반짝반짝';
spans[1].innerHTML = '작은별';
};
<body>
<span></span><br>
<span></span>
</body>
- getElementsByName(name) : name을 탐색하여 문서 객체의 배열 반환
window.onload = function(){
var prev = document.getElementsByName('prev');
var next = document.getElementsByName('next');
prev[0].innerHTML = '이전';
next[0].innerHTML = '다음';
var country = document.getElementsByName('seasons');
country[3].checked = true; // 체크
var content = document.getElementsByName('content');
content[0].value='흰 눈이 소복소복';
};
<body>
<button name="prev">prev</button><br>
<button name="next">next</button><br>
<input type="checkbox" name="seasons" value="봄">봄
<input type="checkbox" name="seasons" value="여름">여름
<input type="checkbox" name="seasons" value="가을">가을
<input type="checkbox" name="seasons" value="겨울">겨울
<br>
<input type="text" name="content">
</body>
- getElementsByClassName(class) : 클래스명을 탐색하여 문서 객체의 배열 반환
⭐ HTML5 또는 최신 브라우저에서 지원
window.onload = function() {
// 전체 태그에서 클래스명으로 탐색
var matched = document.getElementsByClassName('matched');
// 경고창에 태그내용 표출
var output = '';
for(var i=0;i<matched.length;i++){
output += matched[i].innerHTML + '\n';
}
alert(output);
};
window.onload = function() {
var top = document.getElementById('top');
// top 객체의 하위태그에서 클래스명을 탐색
var topMatched = top.getElementsByClassName('matched');
// 경고창에 태그내용 표출
var output='';
for(var i=0;i<topMatched.length;i++){
output += topMatched[i].innerHTML + '\n';
}
alert(output); // A B
};
window.onload = function() {
// 여러 개의 클래스명을 가진 객체 탐색 - 띄어쓰기로 구분, 순서무관
var classes = document.getElementsByClassName('matched unmatched');
// 경고창에 태그내용 표출
var output = '';
for(var i=0;i<classes.length;i++){
output += classes[i].innerHTML + '\n';
}
alert(output); // B
};
<body>
<p id="top">
<span class="matched">A</span>
<span class="matched unmatched">B</span>
<span class="unmatched">C</span>
</p>
<p id="bottom">
<span class="matched">Z</span>
</p>
</body>
'Web > JavaScript' 카테고리의 다른 글
[JavaScript] 이벤트 모델, 이벤트 타입, 이벤트 전파 (0) | 2021.08.18 |
---|---|
[JavaScript] DOM - 태그 생성, 삭제, 스타일 처리 (0) | 2021.08.18 |
[JavaScript] 내장 객체(Built-in Objects) (0) | 2021.08.17 |
[JavaScript] 객체의 종류, BOM(브라우저 객체 모델) (0) | 2021.08.17 |
[JavaScript] 생성자 함수 (0) | 2021.08.17 |